APIs can be used for adding functionality to your WordPress page. Application Programming Interface (API) works as an intermediate between applications for communication and exchanging information in real-time. WordPress is a flexible platform that allows users to create their websites.
So, for the better functionality of the website, you can use third-party API integration & customization. Mostly, a Web API is integrated that uses HTTP to retrieve data anywhere from the internet. Clients can create a custom page template that will display results from API calls. The WordPress theme can be changed by adding the template to the child theme.
Steps to Integrate an External API into WordPress Page:
Third-party APIs can be integrated into WordPress to expand the functionality of your website. APIs can be used with all types of advanced languages. Users get the output data in PHP, JSON, Java, etc.
1. Setup Working Environment:
Start by setting up the working environment for the external API integration & customization into WordPress. Then, users can download the postman app, which provides a suitable domain for API development.
The application makes connecting, testing and creating an API simpler. The postman app is completely free for small teams and individuals. It mainly involves building a widget in a simple WordPress plugin.
2. Code the plugin basics:
Developers have to create a simple plugin called Flickr-widget. Create a Flickr-widget.php file inside a folder named the same as the plugin.
For example, if you want to create an API key for the latest movies on Netflix, the plugin is named Netflix.php file. At the top of the file, place a code using different Authors and URLs.
Place the newly developed plugin folder on your WordPress page: wp-content > plugins. Users can activate it from the WordPress admin dashboard > plugins. The changes are not visible in your WordPress since no functional codes are added.
3. Setup and Test API with Postman:
Users can test API integration & customization in WordPress with Postman. The data is cached with WordPress. We used the Flickr-widget plugin for WordPress. Hence a Flickr REST API is incorporated.
Netflix uses an API, The Next Generation Netflix Global Search, which helps users view more than 100 recently added items on Netflix. Users can connect, collect and format data from Flickr REST API/Netflix REST API with a single click.
The Flickr REST API is used to get, post, or delete any Flickr data users want. The Netflix REST API can be used for viewing the latest movies added to Netflix.
4. Get an API Key for REST API:
Clients need to understand which API they want to use. Then, they can use the key to display up to thousands of images. The price is very much affordable. If you call the API more than 100 times a day, it is mandatory to pay. Users have to create a free account for using a RapidAPI for the first time.
You can create a RapidAPI account for free by clicking the signup button. Users get quick access to over thousands of APIs by subscribing to RapidAPI. Then, you can request an API key. Many API providers have set rules and regulations for using API. APIs have limited access and commercial and non-commercial usage.
5. Make a Child Theme
It is important to have a child theme on your WordPress website.
The steps to create a Child Theme are as follows:
- Create a child theme folder. For example, if your existing theme folder is wp-content/themes/twenty/twenty/, you have to create another folder in the same directory. The folder is named twenty-twenty-child.
- Now, create a stylesheet called style.css for the new child theme folder. This file is required, even if it has only one code. Then, you can clear the cache while making changes.
/*
Theme Name: Twenty Nineteen Child
Template: twentynineteen
Version: 1.0.0
*/
- An Enqueue stylesheet is created in functions.php in the new child theme folder.
<?php add_action( 'wp_enqueue_scripts', 'my_theme_enqueue_styles' ); function my_theme_enqueue_styles() { $parent_style = 'twentynineteen-style'; // This is 'twentynineteen-style' for the Twenty Nineteen theme. wp_enqueue_style( $parent_style, get_template_directory_uri() . '/style.css' ); wp_enqueue_style( 'child-style', get_stylesheet_directory_uri() . '/style.css', array( $parent_style ), wp_get_theme()->get('Version') ); }
Now, activate the child theme. It is done in the WordPress admin area called Appearance>>Themes. Users can capture images and upload them to the child theme folder. The file is saved in screenshot.png format.
6. Create a Custom Template:
After creating a child theme, users are free to add a custom page template for interacting with API integration & customization in WordPress. This step comes after initiating the child theme. You can put together a custom template that will act as the basis of your page for API-based interactions.
The header section is first taken into consideration. Next, a template is created for displaying the recent movies arriving on Netflix or the newest photos uploaded on Flickr.
<?PHP
/*
Template Name :
LatestFlickrImages
*/
The file is saved in a .php format in the child theme folder. A one-page template is added after getting a file name and location, which performs like the parent theme.
Get_header(); ?> <div id=”primary” class=”content-area”> <main id=”main” class=”site-main”> <?php // Start the Loop. While ( have_posts() ) : the_post(); get_template_part( ‘template-parts/content/content’, ‘page’ ); // If comments are open or we have at least one comment, load up the comment template. If ( comments_open() || get_comments_number() ) { comments_template(); } endwhile; // End the loop. ?> </main><!-- #main à </div><!-- #primary à <?php get_footer();
7. Integrate API into your page template
Now, you can proceed to API integration & customization into the template page. The code is used as a starting point to pull out the loop. Then, add the REST API integration. The sample PHP curl code for API is mentioned below.
In the following snippet, users have to replace X’s with their unique API keys.
<?php $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "https://unogsng.p.rapidapi.com/genres", CURLOPT_RETURNTRANSFER => true, CURLOPT_FOLLOWLOCATION => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( "x-rapidapi-host: unogsng.p.rapidapi.com", "x-rapidapi-key: xxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxxx" ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { echo "cURL Error #:" . $err; } else { echo $response; }
Now the results are displayed by decoding the JSON returned from the API. You get an array of objects which are looped into the grid.
The custom classes are mainly used to define the grid. However, it is unnecessary if your website uses other UI frameworks or bootstraps. The page template is displayed by replacing the X’s with the API key.
<?php
/*
Template Name: Latest Netflix Releases
Description: This is a custom page template that will use a third-party API
to pull a list of up to 100 items released on Netflix within the last 7 days.
*/ //This is used to tell the API what we want to retrieve $lastWeek = date("Y-m-d", time()-(24*3600*7)); //Show the header of your WordPress site so the page does not look out of place get_header(); ?> <div id="primary" class="content-area"> <main id="main" class="site-main"> <?php $curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => "https://unogsng.p.rapidapi.com/search?newdate=$lastWeek&orderby=date&audio=english", CURLOPT_RETURNTRANSFER => true, CURLOPT_FOLLOWLOCATION => true, CURLOPT_ENCODING => "", CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 30, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => "GET", CURLOPT_HTTPHEADER => array( "x-rapidapi-host: unogsng.p.rapidapi.com", "x-rapidapi-key: xxxxxxxxxxxxxxxxxxxxxxxxxxxxx" ), )); $response = curl_exec($curl); $err = curl_error($curl); curl_close($curl); if ($err) { if ($debug) echo "cURL Error #:" . $err; else echo "Oops, something went wrong. Please try again later."; } else { //Create an array of objects from the JSON returned by the API $jsonObj = json_decode($response); //Reorder the items so the newest releases are first $newestReleasesFirst = array_reverse($jsonObj->results); //Create a simple grid style for the listings $pageCSS = "<style> .netflix-wrapper{ display:grid; grid-template-columns: 200px 200px 200px; } .show-wrapper{padding:10px;} </style>"; //Create the WordPress page content HTML $pageHTML="<h2>Netflix releases since $lastWeek (in English)</h2>"; $pageHTML.="<div class='netflix-wrapper'>"; //Loop through the API results foreach($newestReleasesFirst as $showObj) { //Put each show into an html structure // Note: if your theme uses bootstrap use responsive classes here $pageHTML.="<div class='show-wrapper'>"; //Not all items have a 'poster', so in that case use the img field if (!empty($showObj->poster)) $thisImg = $showObj->poster; else $thisImg = $showObj->img; //Show the image first to keep the top edge of the grid level $pageHTML.="<img style='max-width:166px;float:left;' src='".$thisImg."' />"; $pageHTML.="<h3>".$showObj->title."</h3>"; $pageHTML.="<span>added to netflix ".$showObj->titledate."</span>"; $pageHTML.="<div style='float:left;'>".$showObj->synopsis."</div>"; $pageHTML.="</div>"; } $pageHTML.="</div>"; echo $pageCSS.$pageHTML; } // end of check for curl error ?> </main><!-- #main --> </div><!-- #primary --> <?php //Show the footer of the WordPress site to keep the page in context get_footer();
8. Add a New Page:
You can add a new page using your new custom page template from the WordPress admin area. Select the desired custom template to add to the new page and external API integration & customization.
Conclusion:
The above article discusses how to use an external API in the WordPress page through codes. The article cited an example of Netflix releases into a WordPress page to better understand the API integration & customization.
The article focuses on incorporating an external API within the custom page template. You can also change the API parameters as per your requirements. The method can integrate any REST API into a WordPress page.
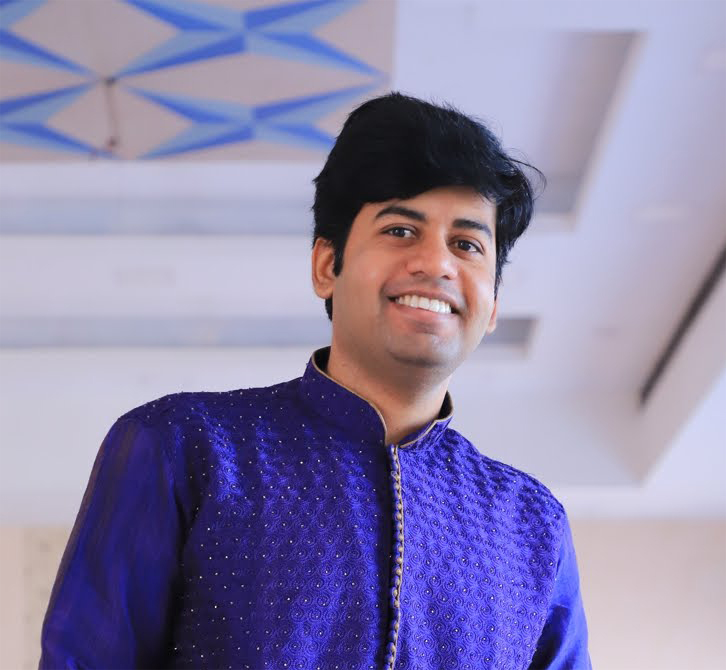
Sonal S Sinha is managing director of Shri Krishna Technologies. He has been writing about website designing, digital marketing and WordPress ecosystem for the past 15+ years. He handles the overall operations and in his free time writes suitable blog posts which are knowledgeable and informative.